How To Make a ScoreBoard in Unity Game Engine With C#
Hello everyone, today post will be a tutorial. In this tutorial i will show you how to make an offline scoreboard in unity game engine using Player Prefs, we will be making some script that handle the Saving and Loading data in the game, we will also make some sorting to the score.
PlayerPrefs or player preferences is a feature that allow you to store data of your game into disk, this is different than a database, PlayerPrefs is basically used to store Game Settings, like your graphic settings. But this time we will use PlayerPrefs to store our Score data. Another alternative is to use SQL Lite, but it's some advanced thing, right now we only need to store our simply score, so PlayerPrefs is fit enough for this.
PlayerPrefs are stored in different location on every different devices.
Editor/Standalone
On Mac OS X PlayerPrefs are stored in ~/Library/Preferences folder, in a file named unity.[company name].[product name].plist, where company and product names are the names set up in Project Settings. The same .plist file is used for both Projects run in the Editor and standalone players.
On Windows, PlayerPrefs are stored in the registry under HKCU\Software\[company name]\[product name] key, where company and product names are the names set up in Project Settings.
On Linux, PlayerPrefs can be found in ~/.config/unity3d/[CompanyName]/[ProductName] again using the company and product names specified in the Project Settings.
On Windows Store Apps, Player Prefs can be found in %userprofile%\AppData\Local\Packages\[ProductPackageId]>\LocalState\playerprefs.dat
On Windows Phone 8, Player Prefs can be found in application's local folder
WebPlayer
On Web players, PlayerPrefs are stored in binary files in the following locations:
Mac OS X: ~/Library/Preferences/Unity/WebPlayerPrefs
Windows: %APPDATA%\Unity\WebPlayerPrefs
There is one preference file per Web player URL and the file size is limited to 1 megabyte. If this limit is exceeded, SetInt, SetFloat and SetString will not store the value and throw a PlayerPrefsException.
The Beggining of The Scripting
Let's start our scripting by making a variable first, after you create a C# Script, in this case i will name my script as ScoreBoard, open the script and the then we gonna modify the default script template,
public class ScoreBoard : MonoBehaviour
{
static ScoreBoard scoreBoard;
static string separator = "~S~";
// Use this for initialization
void Start ()
{
scoreBoard = this;
}
void Update ()
{
}
}
In the code above, i've add two variable, scoreBoard and separator, both of them are static, because i want to use them wherever i want by just calling the ClassName. In the void Start function, i assign ScoreBoard object (component in inspector) to the static field scoreBoard, so i have a refference to them.
Having a static variable that reference to an object is useful when you want to make a static method but you need reference to a non static object.
After that, we now need to add non static variable, so we can control some variable inside the inspector. I add those two variable.
Having a static variable that reference to an object is useful when you want to make a static method but you need reference to a non static object.
After that, we now need to add non static variable, so we can control some variable inside the inspector. I add those two variable.
public int scoreCount = 10;
that variable allow you to control how many score can be saved and loaded,
The SaveScore Method
After all variable and class PlayerScore are ready, now we gonna make the SaveScoreMethod. We gonna need 2 parameter for this method.
string name //the playername that will save score
int score //the score of the player
The full code will be like this, if you want the complete explanation about what's going on in this method, please check out this video : Unity How To Make Scoreboard English Part 1
public static void SaveScore (string name, int score)
{
List playerScores = new List();
for (int i = 0; i < scoreBoard.scoreCount; i++)
{
if (PlayerPrefs.HasKey("Score" + i))
{
string[] scoreFormat = PlayerPrefs.GetString("Score" + i).Split(new string[] { separator }, System.StringSplitOptions.RemoveEmptyEntries);
playerScores.Add(new PlayerScore(scoreFormat[0], int.Parse(scoreFormat[1])));
} else
{
break;
}
}
if (playerScores.Count < 1)
{
PlayerPrefs.SetString("Score0", name + separator + score);
return;
}
playerScores.Add(new PlayerScore(name, score));
playerScores = playerScores.OrderByDescending(o => o.playerScore).ToList();
for (int i = 0; i < scoreBoard.scoreCount; i++)
{
if (i >= playerScores.Count) { break; }
PlayerPrefs.SetString("Score" + i, playerScores[i].GetFormat());
}
}
Here's the video version
Continue Reading in Part 2
ABOUT THE AUTHOR
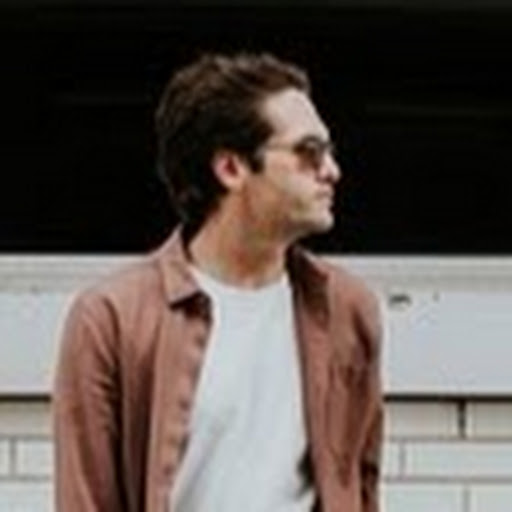
Hello We are OddThemes, Our name came from the fact that we are UNIQUE. We specialize in designing premium looking fully customizable highly responsive blogger templates. We at OddThemes do carry a philosophy that: Nothing Is Impossible
I can see that you are an expert at your field! I am launching a website soon, and your information will be very useful for me.. Thanks for all your help and wishing you all the success in your business. etcgame
ReplyDelete